Database¶
We support Oracle and Postgres databases.
Multiple hosts¶
The dsn parameter of service database config should be specified to enable multihost option for Postgres.
DSN may be specified in the following format: luna:luna@postgres01:5432,postgres02:5432/luna_faces?some_option=some_value. It is possible to partially fill in the DSN string (e.g. postgres01,postgres02/luna_faces), and then the missing parameters will be filled in from proprietary parameter values (or defaults).
At startup, the service will create a pool of connections to one of DSN hosts available. In case of problems with establishing a connection after several unsuccessful attempts, the service will again try to set up a connection pool to any of DSN hosts available.
DB scheme.¶
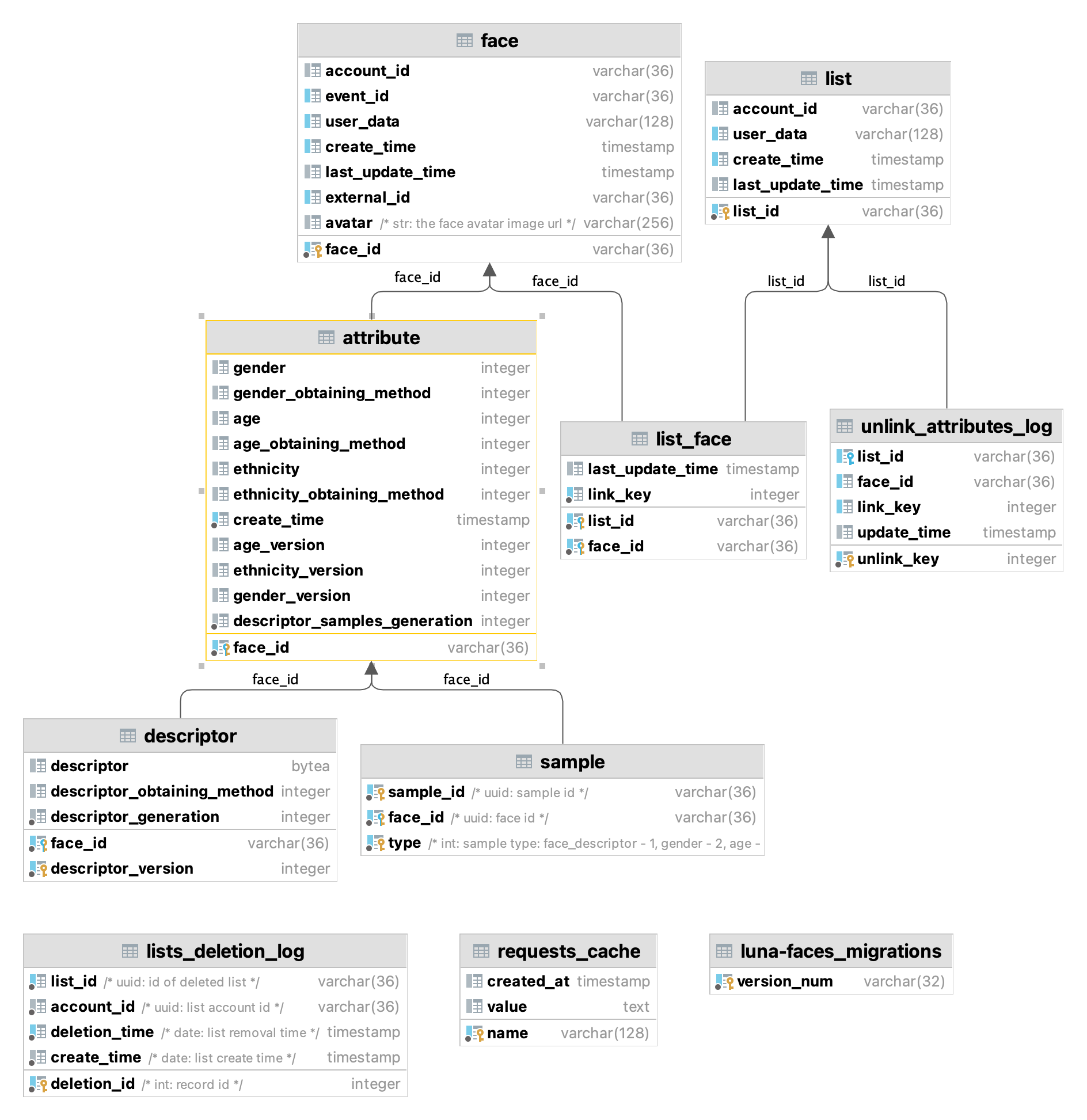
- class luna_faces.db.faces_db_tools.models.faces_models.Attribute(**kwargs)[source]¶
Database table model for attributes.
- age¶
age
- Type:
int
- age_obtaining_method¶
how the gender was obtained
- Type:
int
- age_version¶
age version
- Type:
int
- create_time¶
date and time of creating attributes
- Type:
DateTime
- descriptor_samples_generation¶
descriptor samples generation
- Type:
int
- ethnicity¶
ethnicity, enum luna_faces.crutches_on_wheels.cow.maps.vl_maps.ETHNIC_MAP
- Type:
int
- ethnicity_obtaining_method¶
how the ethnicity was obtained
- Type:
int
- ethnicity_version¶
ethnicity version
- Type:
int
- face_id¶
face id, uuid.
- Type:
str
- gender¶
gender. 0 - woman, 1- man
- Type:
int
- gender_obtaining_method¶
how the gender was obtained
- Type:
int
- gender_version¶
gender version
- Type:
int
- class luna_faces.db.faces_db_tools.models.faces_models.Column(*args, **kwargs)[source]¶
Release some pretty methods for sqlalchemy.Column.
- class luna_faces.db.faces_db_tools.models.faces_models.Descriptor(**kwargs)[source]¶
Database table model for descriptors.
- descriptor_generation¶
descriptor generation
- Type:
int
- descriptor_obtaining_method¶
how the descriptor was obtained
- Type:
int
- descriptor_version¶
descriptor version
- Type:
int
- face_id¶
face id, uuid.
- Type:
str
- class luna_faces.db.faces_db_tools.models.faces_models.Face(**kwargs)[source]¶
Database table model for faces.
- account_id¶
account uuid in format “xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx”, to which this face belong
- Type:
str
- avatar¶
url to image, that represents the face
- Type:
str
- create_time¶
date and time of creating face
- Type:
DateTime
- external_id¶
external id of the face, if it has its own mapping in external system
- Type:
str
- face_id¶
face id, uuid in format “xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx”
- Type:
str
- last_update_time¶
date and time of last changed of the face
- Type:
DateTime
- user_data¶
client info about the face
- Type:
str
- class luna_faces.db.faces_db_tools.models.faces_models.List(**kwargs)[source]¶
Database table model for lists.
Warning
trg_lists_deletion_log ~— after delete trigger for logging
- account_id¶
account uuid in format “xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx”, to which this list belong
- Type:
str
- create_time¶
date and time of creating list
- Type:
DateTime
- last_update_time¶
date and time of last changed of the list
- Type:
DateTime
- list_id¶
list id, uuid in format “xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx”
- Type:
str
- user_data¶
client info about the list
- Type:
str
- class luna_faces.db.faces_db_tools.models.faces_models.ListFace(**kwargs)[source]¶
Database table model for links between faces and lists.
- face_id¶
face id, uuid in format “xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx”
- Type:
str
- last_update_time¶
date and time of last attach/detach face to list
- Type:
DateTime
- link_key¶
number of link face to list
- Type:
int
- link_key_index = Index('list_id_link_key_index', Column('list_id', String(length=36), ForeignKey('list.list_id'), table=<list_face>, primary_key=True, nullable=False), Column('link_key', Integer(), table=<list_face>, nullable=False, default=Sequence('link_key', metadata=MetaData(bind=None))))¶
index link keys
- list_id¶
list id, uuid in format “xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx”
- Type:
str
- matcher_load_delta_index = Index('matcher_load_delta_index', Column('link_key', Integer(), table=<list_face>, nullable=False, default=Sequence('link_key', metadata=MetaData(bind=None))))¶
index for load delta into matcher
- class luna_faces.db.faces_db_tools.models.faces_models.ListsDeletionLog(**kwargs)[source]¶
Database table model for lists deletions history
After delete trigger trg_lists_deletion_log` (table list) insert a data.
- account_id¶
account uuid in format “xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx”, to which this face belong
- Type:
str
- create_time¶
create time list
- Type:
DateTime
- deletion_id¶
deletion id
- Type:
str
- deletion_time¶
date list removig
- Type:
DateTime
- list_id¶
list id, uuid in format “xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx”
- Type:
str
- class luna_faces.db.faces_db_tools.models.faces_models.MV_LINK(**kwargs)[source]¶
- link_key¶
number of link face to list
- Type:
int
- list_id¶
list id, uuid in format “xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx”
- Type:
str
- class luna_faces.db.faces_db_tools.models.faces_models.MV_LINK_0(**kwargs)[source]¶
- link_key¶
number of link face to list
- Type:
int
- list_id¶
list id, uuid in format “xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx”
- Type:
str
- class luna_faces.db.faces_db_tools.models.faces_models.MV_LINK_1(**kwargs)[source]¶
- link_key¶
number of link face to list
- Type:
int
- list_id¶
list id, uuid in format “xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx”
- Type:
str
- class luna_faces.db.faces_db_tools.models.faces_models.MV_UNLINK(**kwargs)[source]¶
- list_id¶
list id, uuid in format “xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx”
- Type:
str
- unlink_key¶
number of link face to list
- Type:
int
- class luna_faces.db.faces_db_tools.models.faces_models.MV_UNLINK_0(**kwargs)[source]¶
- list_id¶
list id, uuid in format “xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx”
- Type:
str
- unlink_key¶
number of link face to list
- Type:
int
- class luna_faces.db.faces_db_tools.models.faces_models.MV_UNLINK_1(**kwargs)[source]¶
- list_id¶
list id, uuid in format “xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx”
- Type:
str
- unlink_key¶
number of link face to list
- Type:
int
- class luna_faces.db.faces_db_tools.models.faces_models.RequestsCache(**kwargs)[source]¶
Model that provides cached data for various often used requests
- class luna_faces.db.faces_db_tools.models.faces_models.Sample(**kwargs)[source]¶
Database model to store samples.
- face_id¶
parent face id, uuid.
- Type:
str
- sample_id¶
id(uuid) of warp
- Type:
str
- type¶
enum for sample type, check ‘SampleType’ in luna_faces/utils/enums.py
- Type:
int
- class luna_faces.db.faces_db_tools.models.faces_models.UnlinkAttributesLog(**kwargs)[source]¶
Database table model for history attach and detach attributes to lists.
- face_id¶
face id, uuid in format “xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx”
- Type:
str
- link_key¶
number of link face to list
- Type:
int
- list_id¶
list id, uuid in format “xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx”
- Type:
str
- unlink_key¶
number of unlink face to list
- Type:
int
- unlink_key_index = Index('list_id_unlink_key_index', Column('list_id', String(length=36), ForeignKey('list.list_id'), table=<unlink_attributes_log>), Column('unlink_key', Integer(), table=<unlink_attributes_log>, primary_key=True, nullable=False, default=Sequence('unlink_key', metadata=MetaData(bind=None))))¶
index unlink keys
- update_time¶
date and time of detach attributes from list
- Type:
DateTime